A thread is a sequence of instructions in a program which can be executed independently of other code. We can also say that thread is subset of a process and a process is made up of many threads.
Threads sometimes called light-weight processes, and they do not require much memory overhead; they are cheaper than processes.
A thread has a starting point, an execution sequence, and a result.
We have a module thread in python. We can easily import it in our program.
syntax:-
import thread
By this module we can easily start a thread.
syntax:-
thread.start_new_thread (function, args,)
Let us see an example to start a thread.
e..g.,
import time import _thread def threadtest(name1, wait): i = 0 while i <= 6: time.sleep(wait) print("Running %s\n" %name1) i = i + 1 print("%s has finished execution" %name1) if __name__ == "__main__": _thread.start_new_thread(threadtest, ("First Thread", 2)) _thread.start_new_thread(threadtest, ("Second Thread", 4)) _thread.start_new_thread(threadtest, ("Third Thread", 6))
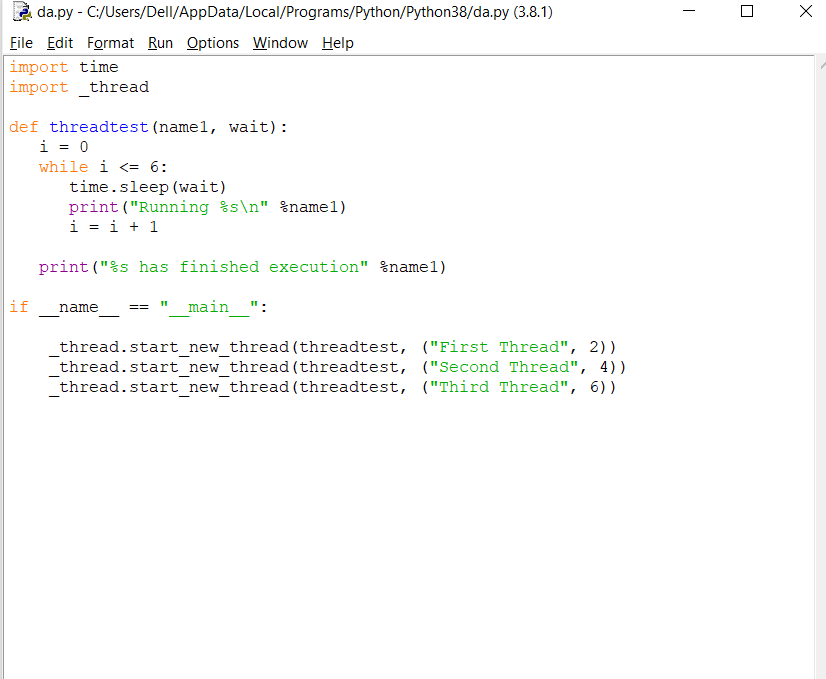

This is all about starting a thread.