How to shuffle a list in python
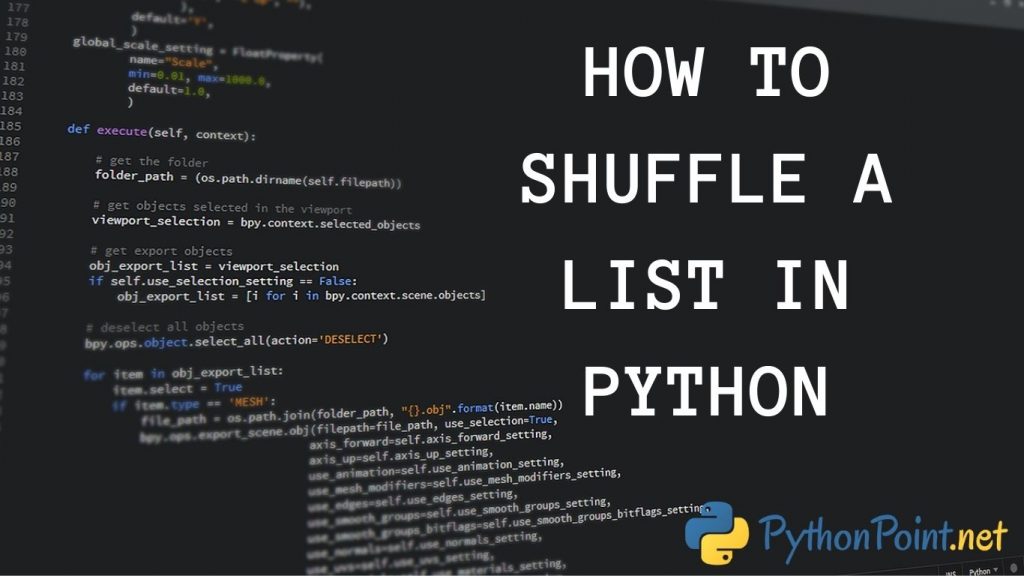
In this article, we will see how to shuffle a list in Python. There are more than one way to achieve this. We will see various methods for shuffling a list
- Using
random.shuffle()
In the random library of Python, there is an inbuilt function random.shuffle()
that in-place shuffles the list. The drawback of this is that list ordering is lost.
import random test = [1, 5, 3, 8, 2, 9] print("Original List: ", test) random.shuffle(test) print("Shuffled List: ", test)
Output:
Original List: [1, 5, 3, 8, 2, 9] Shuffled List: [3, 8, 9, 1, 5, 2]
2. Using random.sample()
This function is better than the shuffle method. This is useful in cases we want to retain the original list.
import random test = [1, 5, 3, 8, 2, 9] print("Original List: ", test) new = random.sample(test, len(test)) print("Shuffled List: ", new)
Output:
Original List: [1, 5, 3, 8, 2, 9] Shuffled List: [3, 9, 8, 1, 5, 2]
Subscribe
Login
Please login to comment
0 Discussion