What is array in python?

An array is basically a data structure which can hold more than one value at a time. It is a collection or ordered series of elements of the same type.
Arrays can only take a single datatype element.
We can take input of arrays by different methods. Here we’ll study it by seeing examples.
e.g.,
a=int(input("Number of elements in the array:-")) n=list(map(int, input("elements of array:-").strip().split())) print(n)
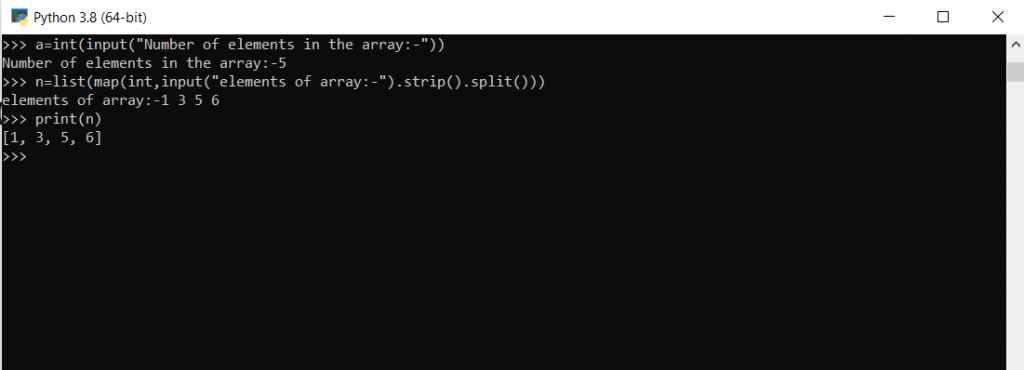
Here we can see in the above example that we have used the map function to take the input of the array from the user.
e.g.,
a=[] n=int(input("Number of elements in array:")) for i in range(0,n): l=int(input()) a.append(l) print(a)

In the above example we have used for loop to get the input of array.
Many of us gets confused whether list and arrays are the same or not.
Lists vs. arrays
- Arrays and lists have the same way of storing data.
- Arrays take only a single data type elements but lists can have any type of data.
- Other than a few operations, the kind of operations performed on them are different.
Basic operations on array:-
- Finding the length of array- Length of an array is the number of elements that are actually present in an array. We can use the function len() to achieve it.
- Adding an element in array- Functions used to add an element to an array are append(), insert() and extend().
- Removing an element- Functions used to remove or delete an element of an array are pop() and remove()
- Array concatenation- Array concatenation is done by using ‘+’ sign as we do in strings.
This is all about arrays in python.
Subscribe
Login
Please login to comment
0 Discussion