How to Remove Punctuation in Python
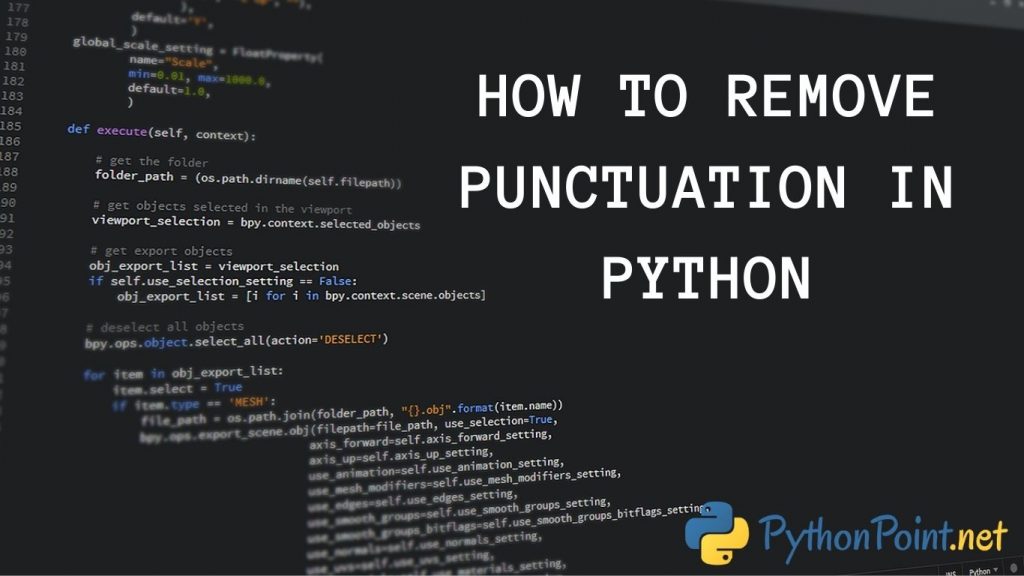
Let’s see how to remove punctuations from string in a python program.
Method 1:
punctuation = '''!()-[]{};:\'",<>./?@#$%^&*_~''' str = "hello world! You are beautiful, <amazing>!" print("Original string: "+str) for i in str: if i in punctuation: str = str.replace(i, "") print("After removing punctuation: "+str)
Output:
Original string: hello world! You are beautiful, <amazing>! After removing punctuation: hello world You are beautiful amazing
In this method we have checked if there is a character in our original string that is a punctuation or not. If punctuation, then that character is replaced by “”
Method 2: Using regex
import re str = "hello world! You are beautiful, <amazing>!" print("Original string: "+str) s = re.sub(r'[^\w\s]', '', str) print("After removing punctuation: "+ s)
Output:
Original string: hello world! You are beautiful, <amazing>! After removing punctuation: hello world You are beautiful amazing
Subscribe
Login
Please login to comment
0 Discussion