How to print reverse string in python?
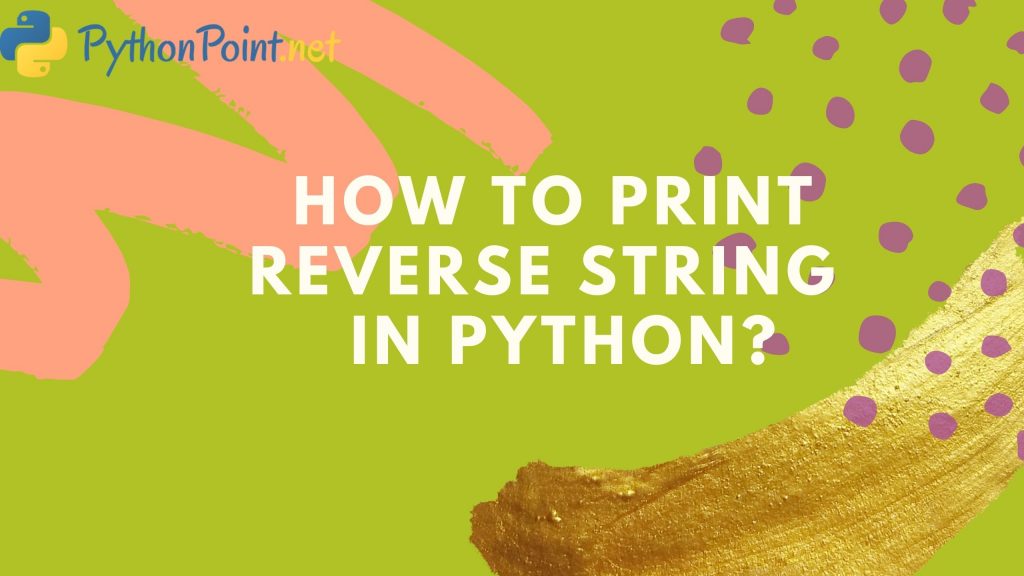
In Python, strings are an ordered sequence of character data. There is no inbuilt method to reverse a string. But strings can be reversed in several ways.
Different Methods
- Loop method– In this method we call a function to reverse a string, which iterates to every element and join each character in the beginning to obtain the reversed string.
E.g.,
s = 'assignment' length = len(s) s_reverse= "" while length>0: s_reverse += s[length-1] length = length-1 print (s_reverse)
Input- assignment Output- tnemngissa
- Slice method– Python’s slicing syntax is used to create a reversed copy of a string. This method offers to put a “step” field as [start, stop, step], and giving no field as start and stop indicates 0 and string length respectively and “-1” denotes starting from end and stop at the start, hence reversing string.
E.g.,
a=”assignment”[: :-1] print(a)
Input- assignment Output- tnemngissa
- Recursion method– In this method, a string is passed as an argument to a recursive function to reverse the string. In the function, the base condition is that if the length of the string is equal to 0, the string is returned. If not equal to 0, the reverse function is recursively called to slice the part of the string except the first character and concatenate the first character to the end of the sliced string.
E.g.,
def reverse(text): if text == "": return text else: return reverse(text[1:]) + text[0] s= "assignment" print (reverse(s))
Input- assignment Output- tnemngissa
- Join method– In this method we use reversed() and this returns the reversed iterator of the given string and then its elements are joined empty string separated using join(). And reversed order string is formed.
E.g.,
string="assignment" print(''.join(reversed(string)))
Input- assignment Output- tnemngissa
This is how we can print reverse string in python.
Subscribe
Login
Please login to comment
0 Discussion