How to Iterate Dictionary in Python
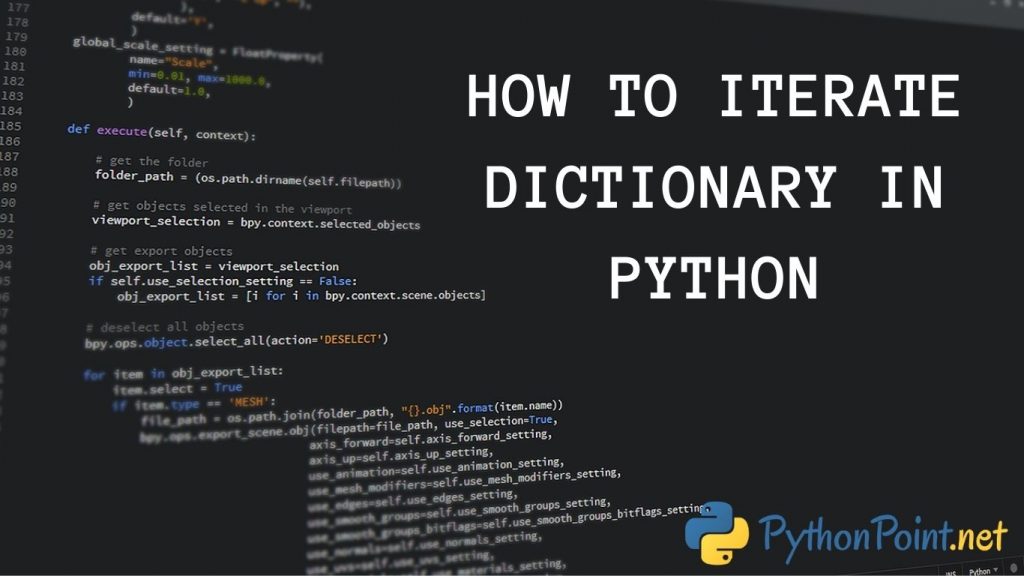
In Python, Dictionary is an unordered collection of data values. Dictionary holds key:value
pair.
To iterate through a dictionary there are several ways:
- Iterate through all keys
- Iterate through all values
- Iterate through all key, value pairs
Iterate through all keys
groupsAndLeaders = { 'Gryffindor' : 'Abhi', 'Ravenclaw' : 'Chandh', 'Hufflepuff' : 'David', 'Slytherin' : 'Harry' } print("List of student groups:\n") for groupnames in groupsAndLeaders: print(groupnames)
Output:
List of student groups: Gryffindor Ravenclaw Hufflepuff Slytherin
Iterate through all values
groupsAndLeaders = { 'Gryffindor' : 'Abhi', 'Ravenclaw' : 'Chandh', 'Hufflepuff' : 'David', 'Slytherin' : 'Harry' } print("List of student leaders:\n") for leaders in groupsAndLeaders.values(): print(leaders)
Output:
List of student leaders: Abhi Chandh David Harry
Iterate through all key, value pairs
groupsAndLeaders = { 'Gryffindor' : 'Abhi', 'Ravenclaw' : 'Chandh', 'Hufflepuff' : 'David', 'Slytherin' : 'Harry' } print("List of student groups and leaders:\n") for group, leader in groupsAndLeaders.items(): print(group, ":", leader)
Output:
List of student groups and leaders: Gryffindor : Abhi Ravenclaw : Chandh Hufflepuff : David Slytherin : Harry
Subscribe
Login
Please login to comment
0 Discussion