How to find Substring in a String in Python
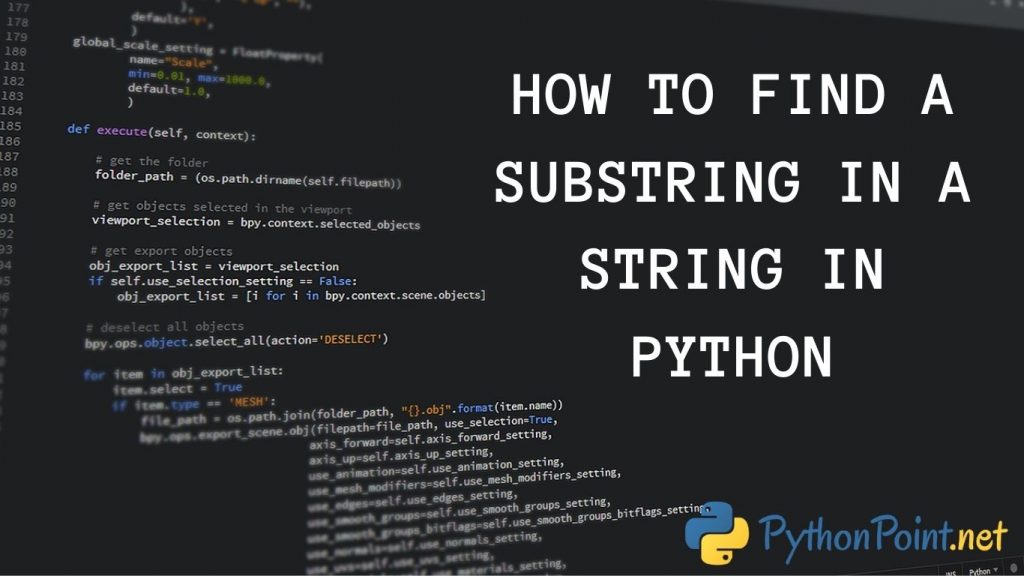
In this article, we will learn to find a substring in a string in Python.
This can be done using the built-in Python method find()
.
The find() method returns the lowest index of the substring if it is found in given string. If its is not found then it returns -1.
Syntax : str.find(sub,start,end)
- sub : It’s the substring which needs to be searched in the given string.
- start : Starting position where sub is needs to be checked within the string.
- end : Ending position where suffix is needs to be checked within the string.
test = 'Python is so cool' print('Substring "Python" found at index: ', test.find('Python')) print('Substring "cool" found at index: ', test.find('cool')) if (test.find('and') == -1): print("Doesn't contain 'and' substring") else: print("Substring 'and' is at index:", test.find('and'))
Output:
Substring "Python" found at index: 0 Substring "cool" found at index: 13 Doesn't contain 'and' substring
Subscribe
Login
Please login to comment
0 Discussion